Fedora Workstation uses GNOME Shell by default and this one was mainly written in JavaScript. JavaScript is famous as a language of front-end development but this time we’ll show its usage for back-end.
We’ll implement a new API using the following technologies: JavaScript, Express and Fedora Workstation. A web browser is being used to call the service (eg. Firefox from the default Fedora WS distro).
Installing of necessary packages
Check: What’s already installed?
$ npm -v $ node -v
You may already have both the necessary packages installed and can skip the next step. If not, install nodejs:
$ sudo dnf install nodejs
A new simple service (low-code style)
Let‘s navigate to our working directory (work) and create a new directory for our new sample back-end app.
$ cd work $ mkdir newApp $ cd newApp $ npx express-generator
The above command generates an application skeleton for us.
$ npm i
The above command installs dependencies. Please mind the security warnings – never use this one for production.
Crack open the routes/users.js
Modify line #6 to:
res.send(data);
Insert this code block below var router:
let data = { '1':'Ann', '2': 'Bruno', '3': 'Celine' }
Save the modified file.
We modified a route and added a new variable data. This one could be declared as a const as we didn‘t modify it anywhere. The result:
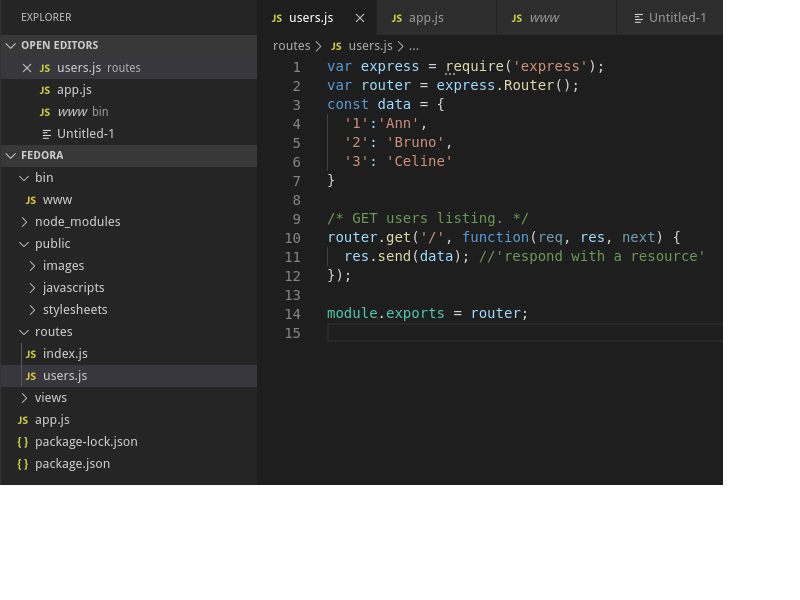
Running the service on your local Fedora workstation machine
$ npm start
Note: The application entry point is bin/www. You may want to change the port number there.
Calling our new service
Let‘s launch our Firefox browser and type-in:
http://localhost:3000/users
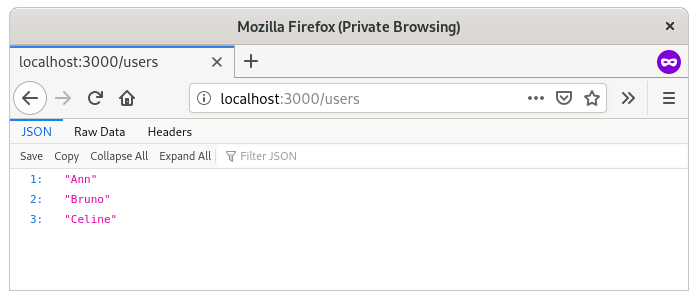
It‘s also possible to leverage the Developer tools. Hit F12 and in the Network tab, select the related GET request and look at the side bar response tab to check the data.
Conclusion
Now we have got a service and and an unnecessary index accessible through localhost:3000. To get quickly rid of this:
- Remove the views directory
- Remove the public directory
- Remove the routes/index.js file
- Inside the app.js file, modify the line 37 to:
res.status(err.status || 500).end(); - Remove the next line res.render(‘error’)
Then restart the service:
$ npm start